How Does It Work With Next.js?
Transcript
[00:00] Let's have a quick look at how you connect Prismic to a Next.js project. There's no need to do anything in your local project just yet.
[00:08] A basic hardcoded title in React might look like this:
<h1>Share team inboxes</h1>
[00:11] But since this content will be dynamically populated from Prismic, you instead use a placeholder for that content, which is replaced later by what Mr McDonald enters in Prismic. This is what we call an API ID. So your title might look like this:
<h1>{ page.data.title }</h1>
[00:25] The API ID is a reference to a specific piece of content or 'field' coming from the Prismic content API.
[00:31] The content API is what makes it possible for Prismic content to live in the cloud and be created in a separate application from the website project. You, the developer, can query the content Mr McDonald creates in his Prismic repository by hitting the API endpoint.
[00:46] To do this in your project, you perform a query in your Next.js page files to the Prismic API using Prismic's JavaScript client.
import * as prismic from '@prismicio/client';
import fetch from 'node-fetch';
export default async function Home() {
const client = prismic.createClient('query-tester', { fetch });
const page = await client.getByUID('author', 'james');
return <h1>{ page.data.title }</h1>;
}
[00:53] The content API contains all Mr McDonald's data formatted as JSON.
{
"id": "XyLKLRAAACsAuKSj",
"uid": "home",
"type": "home_page",
"data": {
"title": "My Name is Bert!",
}
},
[00:57] Using this common format you can reference the content in the code of the farming startups components and pages.
Main takeaways
- API ID: Content reference in Prismic repository
- Prismic Repository: Where Mr McDonald adds his content.
- API endpoint: When the content is returned as JSON.
- Content needs to be queried from the API using Prismic’s Javascript client.
- The Content is returned as JSON.
Practice Activity
Replace the text in the H1 tag with page.data.title
to update the content using JSON that’s queried on this page. Click command ⌘ + s to save your changes and click refresh.
Answer to continue
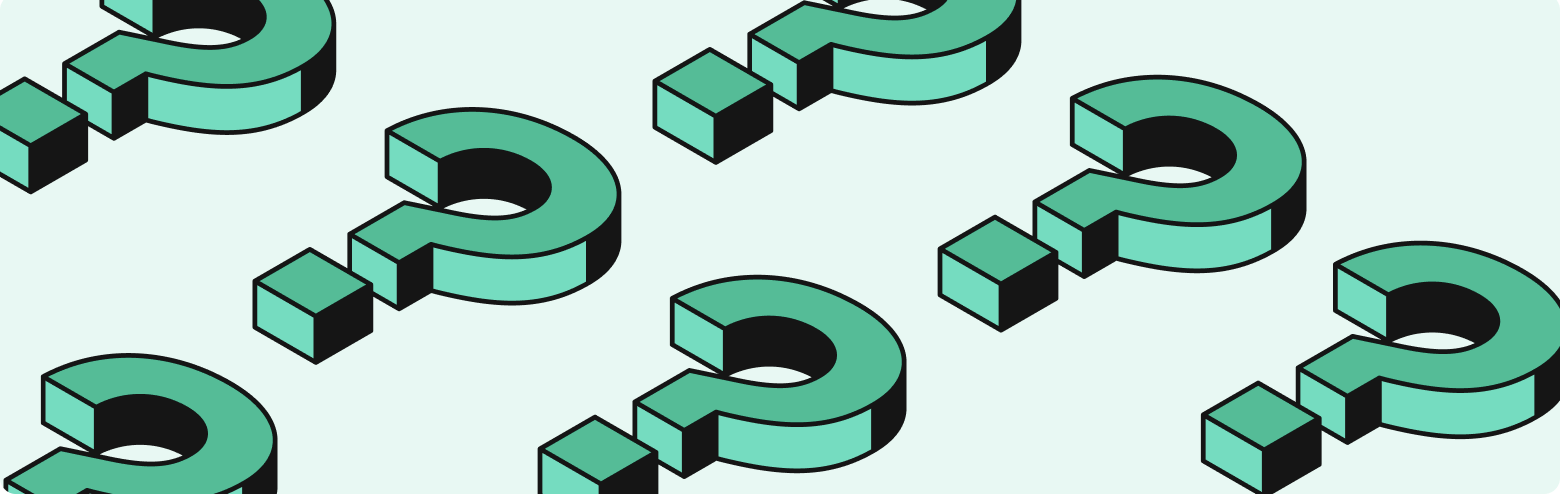
What does the API deliver?