Dynamic Routes and Queries
Transcript
[00:00] Querying content for reusable types is different than querying single types like the homepage. The homepage type has one local file. The content is queried once, and the URL is determined by the homepage files position in the pages directory.
[00:12] For reusable types, you will query multiple documents and therefore will have multiple URLs. The way to handle this is by using dynamic routes. In next.js, you can add brackets to a folder [param] to create a dynamic route.
[00:25] So for our 'marketing_page' type, create a new folder in the app directory called [uid] inside square brackets and inside, create a file called page.jsx. The folder name placeholder will be replaced by the content of the UID field from Prismic. But how does the Next.js application know what the content of the UID field is? Simple, Next.js will statically pre-render all the paths by using generateStaticParams. We use generateStaticParams to do a query for all the documents of a type.
export async function generateStaticParams() {
const client = createClient();
const pages = await client.getAllByType("marketing_page");
return pages.map((page) => {
return { uid: page.uid };
});
}
[00:54] The generateStaticParams function maps through the pages array that is returned by our query, and we then abstract and return the UID property. Now Next.js can get all the file names needed to build the URLs for the routing and the UIDs, you'll need to query your content.
[01:08] Again, the querying is different than the homepage, but not by much. The client is called again, but this time we're querying the Prismic content API with the helper function getByUID. This function accepts a page type API ID as a string and a UID as a string. So we want the 'marketing_page' type and the UID for each page will be passed from the params. Thanks to the query in generateStaticParams.
export default async function Page({ params }) {
const client = createClient();
const page = await client
.getByUID("marketing_page", params.uid)
.catch(() => notFound());
return <SliceZone slices={page.data.slices} components={components} />;
}
[01:31] Now Next.js will query all available marketing page documents from Prismic using the UIDs from generateStaticParams.
[01:37] Templating, and rendering the content on this page is done just the same as the homepage, thanks to the Slice Zone. Thankfully, all the necessary coding for this page type has already been done for you!
[01:45] In Slice Machine, you can click the 'Page Snippet' button to reveal the suggested code for this page. Just click the copy button and paste the code. Enter the page. jsx file in the [uid] folder.
[01:54] How fast is that?! All the code already done for you? Sick.
[01:57] Just save your work and see your new pages at http://localhost:3000/smart-seeds and http://localhost:3000/new-tractor-tech.
Main takeaways
- Create a new file at
app/[uid]/page.jsx
. - Paste the suggested page code from Slice Machine into the file.
- Save and view your new pages at http://localhost:3000/smart-seeds and http://localhost:3000/new-tractor-tech.
Answer to continue
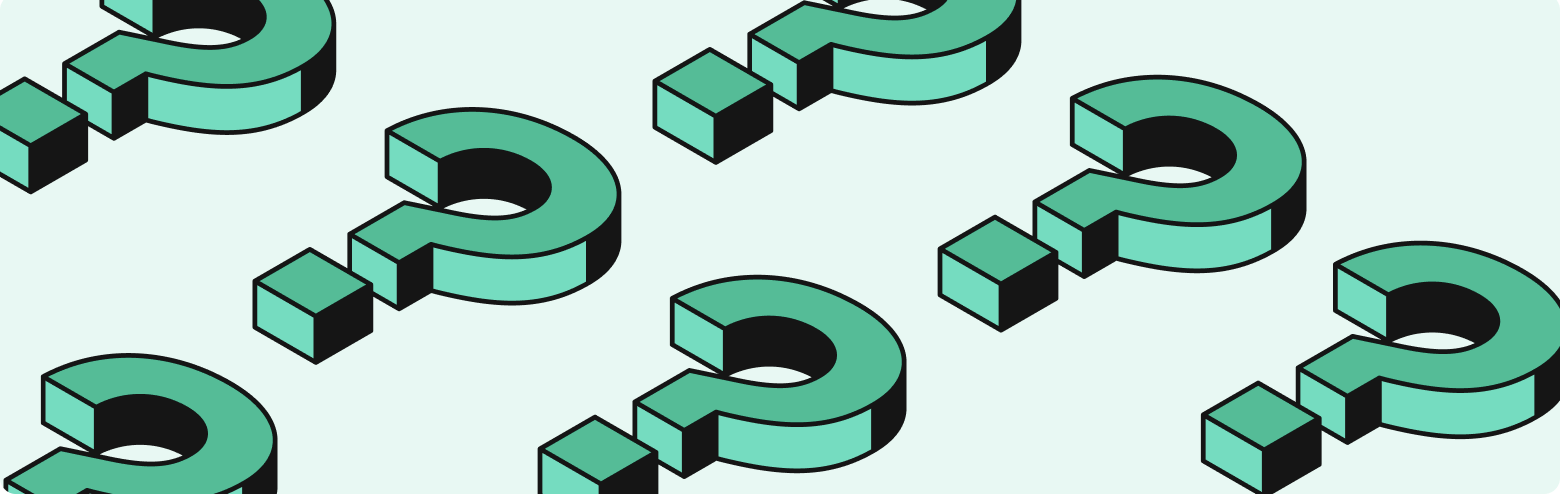
Where does the folder [uid] get it’s value from?
What type of query do we do for the on-page content of reusable page types?