Query the Content and Render the Menu
Transcript
[00:00] Now it's time to code the menu with this content. Luckily, you've already built a React menu just in case. Isn't that good for thinking? I mean, you're the best at this. You'll only need to convert that menu component to get and render the data from the API.
[00:13] So navigate to the components folder and open the Header file and turn the component into an async server component:
export default async function Header() {
[00:28] To get the content. we'll again, import the createClient function:
import { createClient } from '@/prismicio’
[00:31] and call it inside the Header function. Then do a getSingle query for the global_nav type.
const client = createClient()
const nav = await client.getSingle('global_nav')
[00:42] Now you'll have the navigation data available in your Header component. We'll template the title first, which, if you remember, is a Rich Text. So to render it, we'll need to import the Prismic Text helper component from the React package.
import { PrismicText } from '@prismicio/react'
[00:54] Replace the hardcoded company name with the PrismicText component, which gets its content from the nav:
<PrismicText field={nav.data.company_name} />
[01:03] To template the group menu items, we'll use the map function inside the unordered list tag. Each menu item will be output as 'item'. Then for each list item HTML tag, we can stringify the item content to be a unique response for the key attribute which React expects.
<ul className="flex gap-8">
{nav.data.menu_items.map((item) => {
return (
<li key={JSON.stringify(item)}>
</li>
)
})}
</ul>
[01:26] Inside each list item tag, we use Prismic Next link for the menu links and add the key text string for the labels. Import Prismic Next link:
import { PrismicNextLink } from '@prismicio/next'
[01:40] Pass each link to the Prismic link component. Output each label between link tags.
{nav.data.menu_items.map((item) => {
return (
<li key={JSON.stringify(item)}>
<PrismicNextLink field={item.menu_link}>
{item.menu_label}
</PrismicNextLink>
</li>
)
})}
[01:51] In the Next.js Layout file, import the Header component:
import Header from '@/components/Header'
[01:56] then add it inside the body tag, inside the div, which will allow us to pass the fonts.
<body>
<div className={`${inter.variable} ${space_grotesk.variable}`}>
<Header />
{children}
</div>
</body>
[02:01] Save your changes, and marvel at your fully functioning website with a menu built using Prismic and Next.js. Wow! Does anyone else feel like a code wizard here? Or, is it just me?
Answer to continue
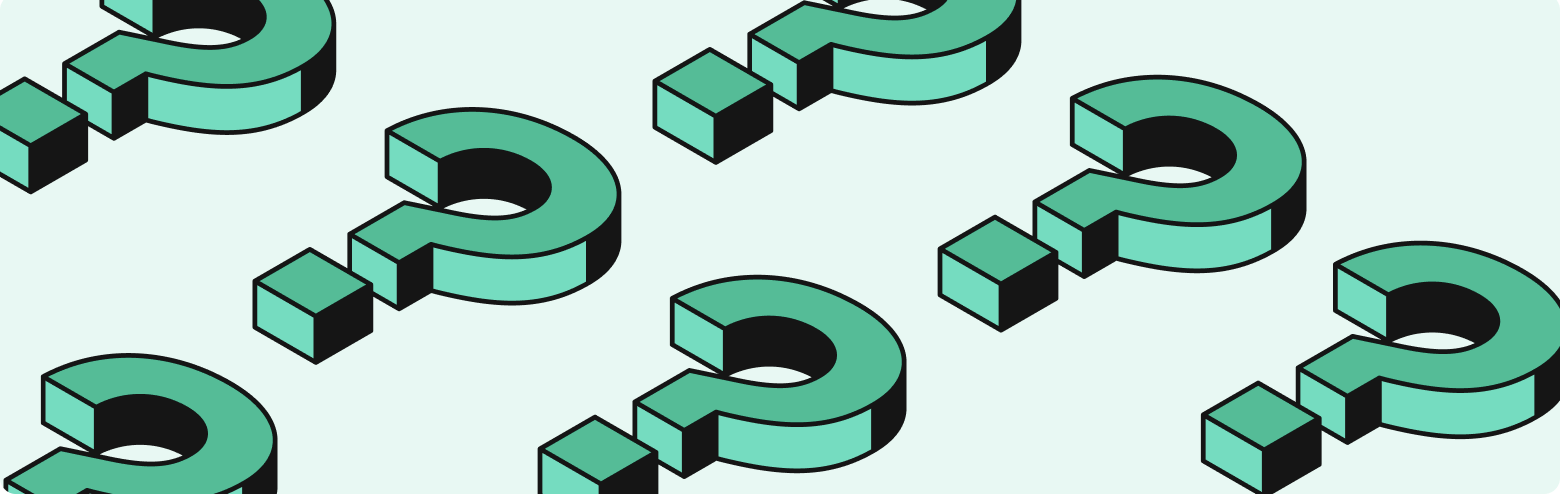
Do all your menu links work?