Configure Previews
Transcript
[00:00] Now we're going to focus on setting up Prismic previews in your Next.js project. This feature will enable you to view your content as it would appear on your website before publishing it. This brings a whole host of benefits, including:
[00:11] - providing a valuable opportunity for final checks and edits in isolation from the live site.
[00:16] - testing on different devices.
[00:18] - sharing potential changes with other stakeholders, even if they don't have access to Prismic.
[00:23] - Saving costs on build times as you don't need to rebuild the live website every time you want to do a check.
[00:30] This is done by layering cookies on top of your website project so that you see the content in your and only your browser totally independent of any other users. So our client, Mr. McDonald, can test his changes on the homepage and show it to Mrs. McDonald so she can make sure the website users never see his crazy content changes.
[00:50] Remember that in an earlier lesson, the Prismic Next package was installed by the Slice Machine setup command. If you haven't already followed that lesson, I highly recommend you do so to get set up.
[01:01] First, we'll need to add the Prismic Preview component from the Prismic Next package in your app's layout file, this component requires your repo name from the prismicio file. Here's how to do it:
import { Inter, Space_Grotesk } from 'next/font/google'
import '@/styles/tailwind.css'
import { PrismicPreview } from '@prismicio/next'
import { repositoryName } from '@/prismicio'
import Header from '@/components/Header'
const inter = Inter({
subsets: ['latin'],
variable: '--font-inter',
})
const space_grotesk = Space_Grotesk({
weight: ['600'],
subsets: ['latin'],
variable: '--font-space-grotesk',
})
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<div className={`${inter.variable} ${space_grotesk.variable}`}>
<Header />
{children}
<PrismicPreview repositoryName={repositoryName} />
</div>
</body>
</html>
)
}
[01:26] Next, we will set up the preview routes in your app's API. We'll create two routes. First, api/preview/route.js,
import { draftMode } from "next/headers";
import { redirectToPreviewURL } from "@prismicio/next";
import { createClient } from "@/prismicio";
export async function GET(request) {
const client = createClient();
draftMode().enable();
await redirectToPreviewURL({ client, request });
}
[01:40] which starts the Next.js draft mode, and handles the redirection from Prismic to your preview documents webpage. Then api/exit-preview /route.js,
import { exitPreview } from "@prismicio/next";
export async function GET() {
return await exitPreview();
}
[01:54] which ends the preview session and clears the cookies from your browser.
[01:59] Push your changes to your GitHub repository to update your live website.
[02:04] Finally, let's head back to your Prismic repository and configure the previews there. Go to Settings, Previews, manage your previews. You can ignore the step about including the Prismic toolbar as the Prismic Preview component we added earlier handles that for us.
[02:20] Fill out the fields with the following information. For domain, enter the domain of your live website deployed on Vercel. And for Preview Route, enter /api/preview. And there you have it.
/api/preview
[02:32] You've successfully set up Prismic previews in your Next.js project. Now you can conveniently preview your content in the context of your website before it goes live. We'll try that in the next screen.
Summary
- Add the
PrismicPreview
component to the layout file. - Add preview and exit-preview routes to the app's API.
- Configure the preview settings in the repo.
Useful Links
Read more about Next.js Draft mode
Answer to continue
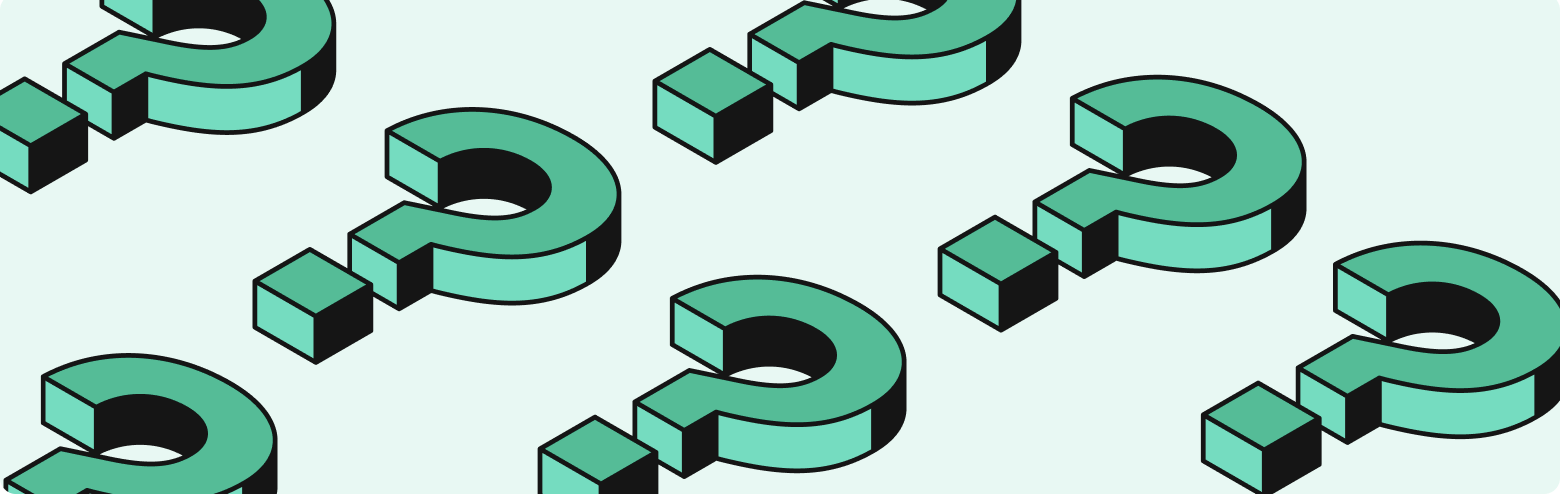
What does the 'exit-preview' route do?