Query Your Homepage Content
Transcript
[00:00] So armed with your newfound knowledge of the client package, you're going to query the content for your homepage. So make sure your project is running on the dev server.
[00:08] In the practice activity on the previous screen we used the createClient function from the library to initialize an instance of the Prismic client and passed it the name of the repository as an option.
[00:18] But doing that every time would be annoying, and we like to be lazy by being smart. So this has already been done for you in the prismicio file.
[00:25] This file gets the repository name from the Slice Machine config file. This is all configured for you by the Slice Machine setup command.
[00:34] The prismicio file also declares the routes for your pages here. Remove the page type path from here since we don't have one yet.
[00:42] So in your project, open the page.jsx file at the base of the 'app' directory and import the createClient function,
import { createClient } from '@/prismicio'
[00:50] and then create a function called queryHomepage; inside that function, call the client as a variable. Then use a query helper to get all the data for your homepage. We can use the getSingle function, which queries a singleton document from the Prismic repository for a specific page type. To do that, declare the API ID of the page type homepage.
const queryHomepage = () => {
const client = createClient()
return client.getSingle('homepage')
}
[01:14] We do this inside a reusable function so we can get the homepage data twice on the page. To call that function in the default page component, we have to use Async / Await in the file.
[01:24] So change the home functional component to include an async declaration, and that's the async part done. Then inside the function, we have to do the await part.
export default async function Home() {
const page = await queryHomepage()
[01:33] Now we need to change the metadata object to a generateMetadata function so that it can query the homepage content. You can read more about this in the Next.js documentation. So change export const metadata to export async function generateMetadata and add the await keyword for the queryHomepage function, in a variable called page. And return the metadata fields.
export async function generateMetadata() {
const page = await queryHomepage()
return {
title: 'EIE - Engineering Intelligent Environments',
description:
'Farming solutions start-up that aims to revolutionize the way farmers work.',
}
}
[01:58] To confirm you've got your content, add a console log on the page variable inside the Home() component function,
export default async function Home() {
const page = await queryHomepage()
console.log(page)
[02:05] and make sure the dev server is running to see your data as JSON in your terminal. You may need to refresh the page in your browser.
[02:12] And boom, you've just got your homepage data as JSON, you're getting good at this, I'm telling you!
Summary
- Remove the page route from the prismicio file
- Import the createClient function in the app/page.jsx file
- Create a queryHomepage function
- Call the function in the home async component
- Change the metadata object to a generateMetadata function and call the homepage query
- Console log the page variable
Read more about generateMetadata in the Next.js docs.
Full Homepage Code
import { createClient } from '@/prismicio'
const queryHomepage = () => {
const client = createClient()
return client.getSingle('homepage')
}
export async function generateMetadata() {
const page = await queryHomepage()
return {
title: 'EIE - Engineering Intelligent Environments',
description:
'Farming solutions start-up that aims to revolutionize the way farmers work.',
}
}
export default async function Home() {
const page = await queryHomepage()
console.log(page.data.slices)
return (
<main>
</main>
)
}
Answer to continue
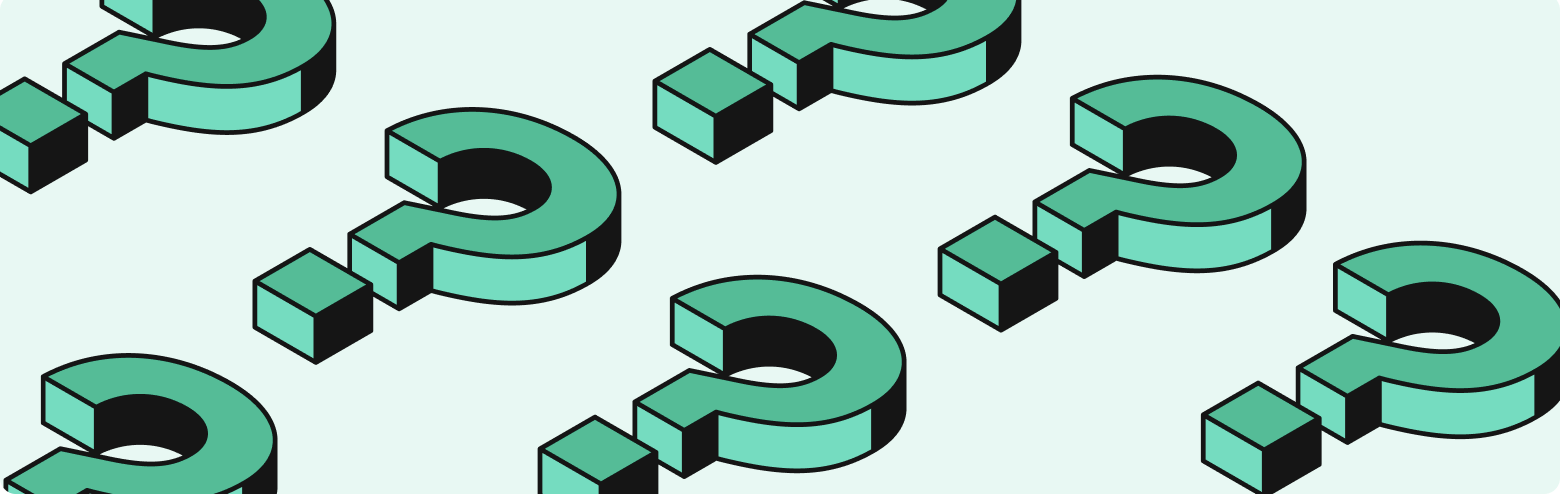
If you console log your page results, can your content as JSON?
Why are we initializing the client in the prismicio.js file?