Template the Static Zone and Simple Fields
Transcript
[00:00] Now lets get down to business with your code. Well start on the Static Zone. If you remember, we have SEO or metadata fields including a title, a description, and an image.
[00:10] Let's use that content on our Next.js projects homepage. The title is a simple field, so we can pass it directly as a string to the Open Graph config in the generate Metadata section of the page using the API ID of page.data .meta_title.
export async function generateMetadata() {
const page = await queryHomepage()
return {
openGraph: {
title: page.data.meta_title,
description: ‘Farming solutions start-up that aims to revolutionize the way farmers work.’
}
}
}
[00:26] The meta description field is a Rich Text field, so therefore it's a structured field and we want to output it as a string for this config.
[00:34] So for that, we can use the asText helper function from the client package. Import everything from this package as the variable prismic.
import * as prismic from '@prismicio/client'
[00:43] Then pass the meta description field to the asText function.
export async function generateMetadata() {
const page = await queryHomepage()
return {
openGraph: {
title: page.data.meta_title,
description: prismic.asText(page.data.meta_description),
}
}
}
[00:54] Voila, our structured text becomes a friendly string ready to join the metadata party.
[00:59] Now let's do the same for the meta image using the asImageSrc helper to create a string from the meta_image structured field.
export async function generateMetadata() {
const page = await queryHomepage()
return {
openGraph: {
title: page.data.meta_title,
description: prismic.asText(page.data.meta_description),
images: prismic.asImageSrc(page.data.meta_image)
}
}
}
[01:10] Remember to save your changes and confirm this has worked for your project by inspecting the code of your homepage in the browser and looking inside the head section. You can then see your metadata and OpenGraph tags.
[01:20] So let's do a little victory dance.
Summary:
- Import everything from the client package as prismic
- Render your SEO content in the
generateMetadata()
function using OpenGraph - Pass the data from the Prismic fields at
page.data.field_api_id
These examples use the Next.js generateMetadata()
method and OpenGraph. For a full list, visit the Next.js docs.
Answer to continue
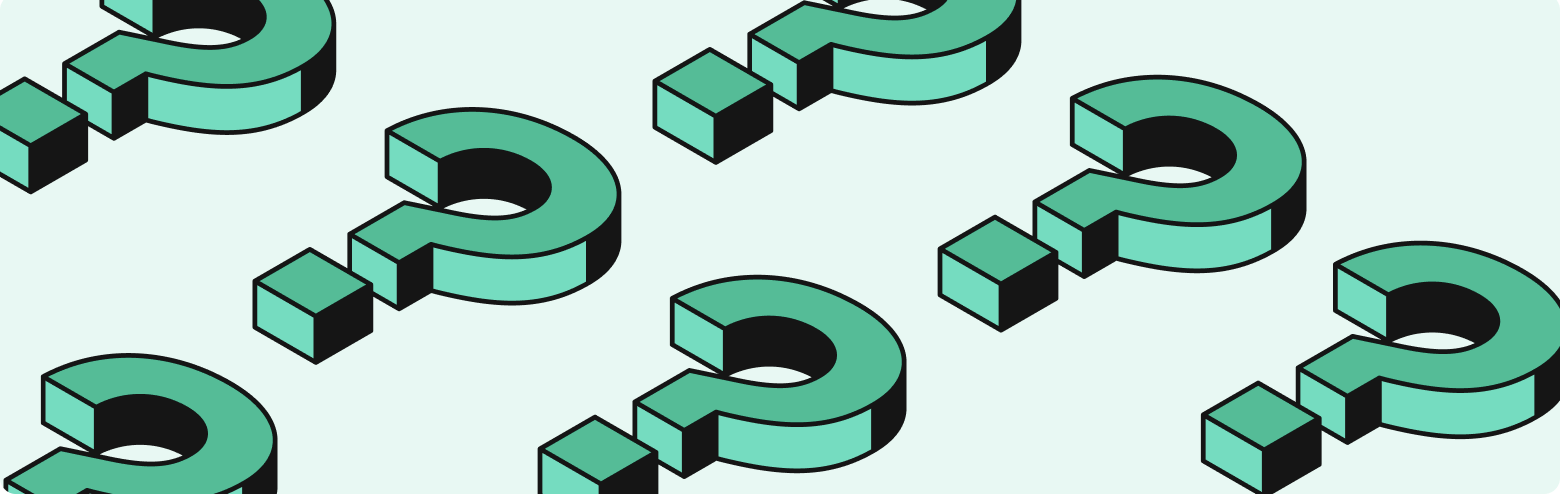
Is your SEO content available?
Confirm that your SEO content was added correctly to the <head> tag by inspecting the page in your browser.